How does the $appends property work in Eloquent Models?
By Tim Wassenburg - 02 Apr, 2023
In Laravel, the Eloquent ORM provides a convenient way to work with your database tables using PHP syntax. One useful feature of Eloquent is the appends property, which allows you to add virtual attributes to your model that aren't actually stored in the database. In this article, we'll explore how appends works in Laravel models and how you can use it to enhance your application.
What is appends?
In Laravel, the appends property is an array that lists the virtual attributes that you want to add to your model. These attributes aren't stored in the database but can be accessed like any other attribute on the model.
For example, let's say you have a Product model with a price attribute stored in the database. You could add a virtual attribute formatted_price to the model using appends. Here's an example:
class Product extends Model
{
protected $appends = ['formatted_price'];
public function getFormattedPriceAttribute()
{
return '$' . number_format($this->price, 2);
}
}
Now, you can access the formatted_price attribute like this:
$product->formatted_price;
When to Use appends
appends is useful when you need to add virtual attributes to your model that are derived from other attributes or logic in your application. For example, you could use appends to add attributes like full_name or age that aren't stored in the database but are calculated based on other attributes like first_name, last_name, and birth_date.
However, it's important to note that using appends can impact the performance of your application, especially if you have many records in your database. This is because every time you retrieve a record from the database, Laravel needs to calculate the value of the virtual attributes, which can be computationally expensive.
Conclusion
The appends property in Laravel models allows you to add virtual attributes to your models that aren't stored in the database. This can be useful for adding calculated attributes to your models, but it's important to be mindful of performance considerations. With appends, you can enhance your application and create more powerful, dynamic models.
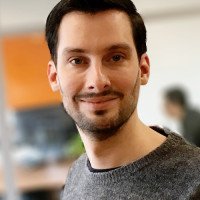
Tim Wassenburg
PHP/Laravel Developer
Other Articles
- Separating Business Logic with The Repository Pattern
- The Laravel Service Container: Dependency Management Made Easy
- The Service Pattern for Clean and Efficient Code
- The difference between composer install and update
- Why using $request->all() is dangerous
- FindaVA Today: A Platform for Connecting Virtual Assistants with Clients
- Laravel Guarded vs Fillable: Choosing the Right Approach
- A Handpicked Collection of the Best Laravel Packages
- InterimBlue by Codexion: Elevate Your Freelance Game