The Service Pattern for Clean and Efficient Code
By Tim Wassenburg - 30 Apr, 2023
In software engineering, the service pattern is a common design pattern that is widely used to provide a layer of abstraction between the business logic and the data access layer of an application. Laravel is a popular PHP framework that makes it easy to implement the service pattern in your application.
The service pattern is particularly useful in Laravel when you want to implement a separation of concerns between the application's logic and its data access layer. By using the service pattern, you can create a layer of abstraction that encapsulates your business logic and provides a standardized interface for accessing data.
Here are some scenarios when you might want to use the service pattern in Laravel:
-
When you have complex business logic: If your application has complex business logic that involves multiple steps and multiple data sources, it can quickly become difficult to manage. By using the service pattern, you can break down your business logic into smaller, more manageable pieces that are easier to understand and maintain.
-
When you need to perform data validation: When you're working with user input, you need to ensure that the data is valid before it's stored in your database. By using the service pattern, you can create a service layer that handles data validation and ensures that only valid data is stored in your database.
-
When you need to interact with external APIs: If your application needs to interact with external APIs, it can be challenging to manage the various endpoints and authentication mechanisms. By using the service pattern, you can create a service layer that abstracts away the details of the external API and provides a standardized interface for accessing it.
-
When you want to implement caching: Caching can significantly improve the performance of your application by reducing the number of queries to your database. By using the service pattern, you can create a service layer that handles caching and ensures that your data is cached appropriately.
To implement the service pattern in Laravel, you can create a directory called "Services" in your application's directory and create a class for each service that you need. Each service class should encapsulate a specific set of business logic and provide a public method for accessing it.
Let's say we have a Product model and we want to create a service function that retrieves all products that are currently on sale. We could create a ProductService class that encapsulates this logic:
class ProductService
{
public function getProductsOnSale(): Collection
{
return Product::query()
->with('media')
->inStock()
->onSale()
->get();
}
}
In this example, we're querying the Product model to retrieve all products that are on sale, are in stock, and eager load media to avoid n+1 queries. We're returning the resulting collection of products.
In our controller, we would inject the ProductService and call its method:
class ProductController extends Controller
{
private $productService;
public function __construct(ProductService $productService)
{
$this->productService = $productService;
}
public function index(): View
{
$productsOnSale = $this->productService->getProductsOnSale();
return view('products.index', compact('productsOnSale'));
}
}
In conclusion, the service pattern is a powerful design pattern that can help you manage complex business logic, implement data validation, interact with external APIs, and implement caching in your Laravel application. By creating a service layer that abstracts away the details
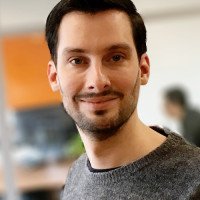
Tim Wassenburg
PHP/Laravel Developer
Other Articles
- Separating Business Logic with The Repository Pattern
- The Laravel Service Container: Dependency Management Made Easy
- The difference between composer install and update
- Why using $request->all() is dangerous
- How does the $appends property work in Eloquent Models?
- FindaVA Today: A Platform for Connecting Virtual Assistants with Clients
- Laravel Guarded vs Fillable: Choosing the Right Approach
- A Handpicked Collection of the Best Laravel Packages
- InterimBlue by Codexion: Elevate Your Freelance Game