Separating Business Logic with The Repository Pattern
By Tim Wassenburg - 16 Apr, 2023
In modern software development, one of the most important design patterns is the Repository Pattern. It's a widely-used pattern that helps developers create a separation of concerns between an application's business logic and data access layer. Laravel, one of the most popular PHP frameworks, supports this pattern and provides built-in functionality for implementing it. In this article, we'll explore the Repository Pattern in Laravel and discuss its benefits.
What is the Repository Pattern?
The Repository Pattern is a design pattern that encapsulates data access logic and provides a standardized interface for accessing data. It separates the business logic from the data access layer, making the application more flexible and easier to maintain. The Repository Pattern also provides a layer of abstraction, allowing developers to change the underlying data storage without affecting the application's business logic.
How does Laravel implement the Repository Pattern?
Laravel has built-in support for the Repository Pattern through its Eloquent ORM. Developers can create a Repository layer that encapsulates data access logic and provides a standardized interface for accessing data. This interface can be used by other layers of the application, such as the Service layer, to access data without worrying about the underlying implementation details.
To implement the Repository Pattern in Laravel, developers can create a Repository class that extends Eloquent's base Repository class. This new Repository class can then be used to encapsulate the data access logic for a particular model or set of models. Laravel's dependency injection container can be used to instantiate and use the Repository classes in controllers or other services.
Benefits of using the Repository Pattern in Laravel:
- Separation of concerns: The Repository Pattern separates the business logic from the data access layer, making the application more modular and easier to maintain.
- Flexibility: The Repository Pattern provides a layer of abstraction, allowing developers to change the underlying data storage without affecting the application's business logic.
- Testability: The Repository Pattern makes it easier to write unit tests for the business logic, as the data access logic is encapsulated in a separate layer.
- Reusability: The Repository Pattern makes it easier to reuse data access logic across multiple parts of the application.
The Repository Pattern is a powerful design pattern that helps developers create a separation of concerns between an application's business logic and data access layer. Laravel's built-in support for this pattern through its Eloquent ORM makes it easy for developers to implement and enjoy the benefits of a well-structured and maintainable codebase. By using the Repository Pattern in Laravel, developers can create modular, flexible, and testable applications that are easier to maintain and scale over time.
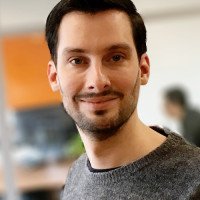
Tim Wassenburg
PHP/Laravel Developer
Other Articles
- The Laravel Service Container: Dependency Management Made Easy
- The Service Pattern for Clean and Efficient Code
- The difference between composer install and update
- Why using $request->all() is dangerous
- How does the $appends property work in Eloquent Models?
- FindaVA Today: A Platform for Connecting Virtual Assistants with Clients
- Laravel Guarded vs Fillable: Choosing the Right Approach
- A Handpicked Collection of the Best Laravel Packages
- InterimBlue by Codexion: Elevate Your Freelance Game